前回は実装するにあたっての準備をしましたが、今回は実際に実装をしていきます
-
-
エラー発生時にメールを送るようにしてみる(M365版)<準備編> - ナストンのまとめ
前回はMailKitを使用してメール送信をしましたが、今回はGraphAPIを使用してメールを送信してみたいと思います ...
続きを読む
認証時に使用するものを用意する
今回のクライアントシークレットトークン認証で使用するものは以下の3つとなります
- クライアントID
- テナントID
- 前回の記事で取得したシークレットトークンの値
Azureから取得する
前回作成したアプリの概要欄上から「クライアントID(アプリケーションID)」と「テナントID(ディレクトID)」が取得できます
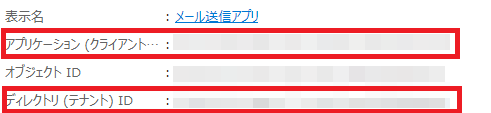
認証オブジェクトを生成する
以下のサイトを参考に実装します
-
-
Microsoft Graph 認証プロバイダーを選択する - Microsoft Graph | Microsoft Learn
アプリケーションにシナリオ固有の認証プロバイダーを選択する方法について説明します。
続きを読む
string[] scopes = { "https://graph.microsoft.com/.default" };
string clientId = "上記の章で取得したクライアントID"
string tenantId = "上記の章で取得したテナントID"
string clientSecret = "前回の記事で取得したシークレットトークンの値"
var options = new ClientSecretCredentialOptions
{
AuthorityHost = AzureAuthorityHosts.AzurePublicCloud,
};
var clientSecretCredential = new ClientSecretCredential(
_tenantId, _clientId, _clientSecret, options);
var client = new GraphServiceClient(clientSecretCredential, _scopes);
実際にメールを送信してみる
メール送信(添付ファイルなし)
以下のサイトを参考に実際にメール送信を実装します
-
-
user: sendMail - Microsoft Graph v1.0 | Microsoft Learn
要求本文に指定されたメッセージを、JSON または MIME 形式で送信します。
続きを読む
public async Task SendMail(string to, string from, string cc, string subject, string text)
{
Microsoft.Graph.Users.Item.SendMail.SendMailPostRequestBody message;
if (cc != "")
{
message = new Microsoft.Graph.Users.Item.SendMail.SendMailPostRequestBody
{
Message = new Message
{
Subject = subject,
Body = new ItemBody
{
ContentType = BodyType.Text,
Content = text
},
ToRecipients = new List<Recipient>
{
new Recipient
{
EmailAddress = new EmailAddress
{
Address = to
}
}
},
CcRecipients = new List<Recipient>
{
new Recipient
{
EmailAddress = new EmailAddress
{
Address = cc
}
}
}
}
};
await client.Users[from].SendMail.PostAsync(message);
}
else
{
message = new Microsoft.Graph.Users.Item.SendMail.SendMailPostRequestBody
{
Message = new Message
{
Subject = subject,
Body = new ItemBody
{
ContentType = BodyType.Text,
Content = text
},
ToRecipients = new List<Recipient>
{
new Recipient
{
EmailAddress = new EmailAddress
{
Address = to
}
}
}
}
};
await client.Users[from].SendMail.PostAsync(message);
}
}
fromは今回作成したAzureアプリと同一テナント上のユーザーである必要があります
メール送信(添付ファイルあり)
次に添付ファイルありのバージョンも以下のサイトを参考に実装してみます
-
-
user: sendMail - Microsoft Graph v1.0 | Microsoft Learn
要求本文に指定されたメッセージを、JSON または MIME 形式で送信します。
続きを読む
public async Task SendMailFileAttach(string to, string from, string cc, string subject, string text, string filePath)
{
var client = GetServiceClient();
Microsoft.Graph.Users.Item.SendMail.SendMailPostRequestBody message;
if(cc != "")
{
message = new Microsoft.Graph.Users.Item.SendMail.SendMailPostRequestBody
{
Message = new Message
{
Subject = subject,
Body = new ItemBody
{
ContentType = BodyType.Text,
Content = text
},
ToRecipients = new List<Recipient>
{
new Recipient
{
EmailAddress = new EmailAddress
{
Address = to
}
}
},
CcRecipients = new List<Recipient>
{
new Recipient
{
EmailAddress = new EmailAddress
{
Address = cc
}
}
},
Attachments = new List<Attachment>
{
new FileAttachment
{
OdataType = "#microsoft.graph.fileAttachment",
Name = Path.GetFileName(filePath),
ContentBytes = File.ReadAllBytes(filePath)
}
}
}
};
await client.Users[from].SendMail.PostAsync(message);
}
else
{
message = new Microsoft.Graph.Users.Item.SendMail.SendMailPostRequestBody
{
Message = new Message
{
Subject = subject,
Body = new ItemBody
{
ContentType = BodyType.Text,
Content = text
},
ToRecipients = new List<Recipient>
{
new Recipient
{
EmailAddress = new EmailAddress
{
Address = to
}
}
},
Attachments = new List<Attachment>
{
new FileAttachment
{
OdataType = "#microsoft.graph.fileAttachment",
Name = Path.GetFileName(filePath),
ContentBytes = File.ReadAllBytes(filePath)
}
}
}
};
await client.Users[from].SendMail.PostAsync(message);
}
}
こちらも先ほどのテキストだけのバージョンとあまり変わらずに実装することができました
最後に
これでM365経由でもメールを送信できるようになりました。フリーアドレス経由 or M365経由でのメール送信を実装してみましたが、状況によって使い分けていければいいもしれません
会社紹介
私が所属しているアドバンスド・ソリューション株式会社(以下、ADS)は一緒に働く仲間を募集しています
会社概要
「技術」×「知恵」=顧客課題の解決・新しい価値の創造
この方程式の実現はADSが大切にしている考えで、技術を磨き続けるgeekさと、顧客を思うloveがあってこそ実現できる世界観だと思っています
この『love & geek』の精神さえあれば、得意不得意はno problem!
技術はピカイチだけど顧客折衝はちょっと苦手。OKです。技術はまだ未熟だけど顧客と知恵を出し合って要件定義するのは大好き。OKです
凸凹な社員の集まり、色んなカラーや柄の個性が集まっているからこそ、常に新しいソリューションが生まれています
ミッション
私たちは、テクノロジーを活用し、業務や事業の生産性向上と企業進化を支援します
-
-
アドバンスド・ソリューション株式会社(ADS)
Microsoft 365/SharePoint/Power Platform/Azure による DX コンサル・シス ...
サイトへ移動